Python 3 Regex Cheat Sheet
Python Home
Introduction
Running Python Programs (os, sys, import)
Modules and IDLE (Import, Reload, exec)
Object Types - Numbers, Strings, and None
Strings - Escape Sequence, Raw String, and Slicing
Strings - Methods
Formatting Strings - expressions and method calls
Files and os.path
Traversing directories recursively
Subprocess Module
Regular Expressions with Python
Regular Expressions Cheat Sheet
Object Types - Lists
Object Types - Dictionaries and Tuples
Functions def, *args, **kargs
Functions lambda
Built-in Functions
map, filter, and reduce
Decorators
List Comprehension
Sets (union/intersection) and itertools - Jaccard coefficient and shingling to check plagiarism
Hashing (Hash tables and hashlib)
Dictionary Comprehension with zip
The yield keyword
Generator Functions and Expressions
generator.send() method
Iterators
Classes and Instances (__init__, __call__, etc.)
if__name__ '__main__'
argparse
Exceptions
@static method vs class method
Private attributes and private methods
bits, bytes, bitstring, and constBitStream
json.dump(s) and json.load(s)
Python Object Serialization - pickle and json
Python Object Serialization - yaml and json
Priority queue and heap queue data structure
Graph data structure
Dijkstra's shortest path algorithm
Prim's spanning tree algorithm
Closure
Functional programming in Python
Remote running a local file using ssh
SQLite 3 - A. Connecting to DB, create/drop table, and insert data into a table
SQLite 3 - B. Selecting, updating and deleting data
MongoDB with PyMongo I - Installing MongoDB ...
Python HTTP Web Services - urllib, httplib2
Web scraping with Selenium for checking domain availability
REST API : Http Requests for Humans with Flask
Blog app with Tornado
Multithreading ...
Python Network Programming I - Basic Server / Client : A Basics
Python Network Programming I - Basic Server / Client : B File Transfer
Python Network Programming II - Chat Server / Client
Python Network Programming III - Echo Server using socketserver network framework
Python Network Programming IV - Asynchronous Request Handling : ThreadingMixIn and ForkingMixIn
Python Coding Questions I
Python Coding Questions II
Python Coding Questions III
Python Coding Questions IV
Python Coding Questions V
Python Coding Questions VI
Python Coding Questions VII
Python Coding Questions VIII
Image processing with Python image library Pillow
Python and C++ with SIP
PyDev with Eclipse
Matplotlib
Redis with Python
NumPy array basics A
NumPy Matrix and Linear Algebra
Pandas with NumPy and Matplotlib
Celluar Automata
Batch gradient descent algorithm
Longest Common Substring Algorithm
Python Unit Test - TDD using unittest.TestCase class
Simple tool - Google page ranking by keywords
Google App Hello World
Google App webapp2 and WSGI
Uploading Google App Hello World
Python 2 vs Python 3
virtualenv and virtualenvwrapper
Uploading a big file to AWS S3 using boto module
Scheduled stopping and starting an AWS instance
Cloudera CDH5 - Scheduled stopping and starting services
Removing Cloud Files - Rackspace API with curl and subprocess
Checking if a process is running/hanging and stop/run a scheduled task on Windows
Apache Spark 1.3 with PySpark (Spark Python API) Shell
Apache Spark 1.2 Streaming
bottle 0.12.7 - Fast and simple WSGI-micro framework for small web-applications ...
Flask app with Apache WSGI on Ubuntu14/CentOS7 ...
Selenium WebDriver
Fabric - streamlining the use of SSH for application deployment
Ansible Quick Preview - Setting up web servers with Nginx, configure enviroments, and deploy an App
Neural Networks with backpropagation for XOR using one hidden layer
NLP - NLTK (Natural Language Toolkit) ...
RabbitMQ(Message broker server) and Celery(Task queue) ...
OpenCV3 and Matplotlib ...
Simple tool - Concatenating slides using FFmpeg ...
iPython - Signal Processing with NumPy
iPython and Jupyter - Install Jupyter, iPython Notebook, drawing with Matplotlib, and publishing it to Github
iPython and Jupyter Notebook with Embedded D3.js
Downloading YouTube videos using youtube-dl embedded with Python
Machine Learning : scikit-learn ...
Django 1.6/1.8 Web Framework ...
Python regular expression
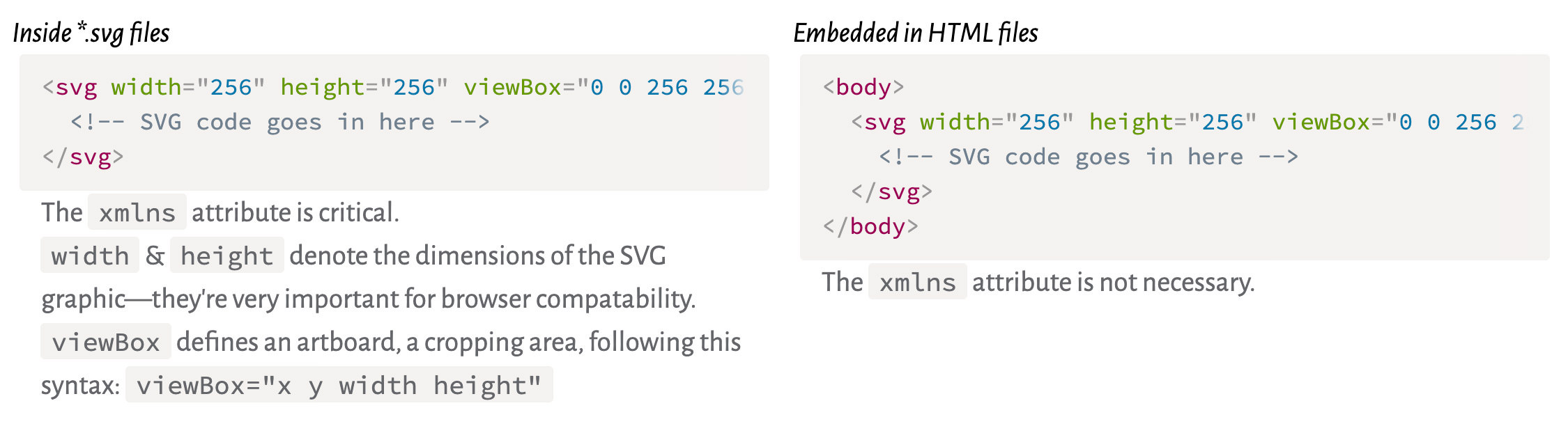
re — Regular expression operations, A regular expression (or RE) specifies a set of strings that matches it; the functions in this module let you check if a particular string matches a given regular Python RegEx ❮ Previous Next ❯ A RegEx, or Regular Expression, is a sequence of characters that forms a search pattern. RegEx can be used to check if a string contains the specified search pattern.
Python Regex Cheatsheet. Regular Expression Basics. Any character except newline: a: The character a: ab: The string ab: a b: a or b: a.: 0 or more a's. Regular Expression Flags; i: Ignore case: m ^ and $ match start and end of line: s. Matches newline as well: x: Allow spaces and comments: L: Locale character classes: u. Python Regular Expression's Cheat Sheet (borrowed from pythex) Special Characters. Escape special characters. Matches any character. ^ matches beginning of string. $ matches end of string. 5b-d matches any chars '5', 'b', 'c' or 'd'. ^a-c6 matches any char except 'a', 'b', 'c' or '6'. R S matches either regex R or regex. S.NET, Python 3, JavaScript: 'whitespace character': any Unicode separator a sb sc a b c D One character that is not a digit as defined by your engine's d D D D ABC. Import re = re.sub(, new, text, count= 0) # Substitutes all occurrences with 'new'. = re.findall(, text) # Returns all occurrences as strings. = re.split(, text, maxsplit= 0) # Use brackets in regex to include the matches. = re.search(, text) # Searches for first occurrence of the pattern. = re.match(, text) # Searches only at the beginning of the.
Regular Expression HOWTO, Regular expressions (called REs, or regexes, or regex patterns) are essentially a tiny, highly specialized programming language embedded inside Python and Most of the standard escapes supported by Python string literals are also accepted by the regular expression parser: a b f n N r t u U v x (Note that b is used to represent word boundaries, and means “backspace” only inside character classes.)
Python RegEx, RegEx Module. Python has a built-in package called re , which can be used to work with Regular Expressions. Import the re module: import re Python has a module named re to work with RegEx. Here's an example: Here's an example: import re pattern = '^as$' test_string = 'abyss' result = re.match(pattern, test_string) if result: print('Search successful.') else: print('Search unsuccessful.')
Regular expression in Python for Beginners
Python regular expressions (RegEx) simple yet complete guide for beginners. In this tutorial, you will learn about regular expressions, called RegExes (RegEx) for short, and use Python's re module to work with regular expressions. RegEx is incredibly useful, and so you must get your head around it early. Regular expressions are the default way of data cleaning and wrangling in Python.
Regular Expressions in Python. Last Updated: August 25, 2020. What is a Regular Expression? It's a string pattern written in a compact syntax, that allows us to quickly check whether a given string matches or …. [Read more] about Regular Expressions in Python.
in string (text) with repl (“good”). import re text = 'Python for beginner is a very cool website' pattern = re.sub ('cool', 'good', text) print text2. Here is another example (taken from Googles Python class ) which searches for all. the email addresses, and changes them to keep the user (1) but have.
Regex tester

regex101: build, debug and share regex, Online regex tester with syntax highlighting for PHP/PCRE, Python, Golang, JavaScript. Contextual help, quiz, cheat sheet, and searchable community patterns. Regex Tester is a tool to learn, build, & testRegular Expressions (RegEx / RegExp). Results update in real-timeas you type. Roll overa match or expression for details. Save& shareexpressions with others.
Regex Tester and Debugger Online, Regular expression tester with syntax highlighting, PHP / PCRE & JS Support, contextual help, cheat sheet, reference, and searchable community patterns. RegExr is an online tool to learn, build, & test Regular Expressions (RegEx / RegExp). Supports JavaScript & PHP/PCRE RegEx. Results update in real-time as you type. Roll over a match or expression for details.
RegExr: Learn, Build, & Test RegEx, Online regular expression tester for Python, PHP, Ruby, JS, Java and MySQL. Regex visualizer. Syntax highlighting. Cheatsheet. Generate string corresponding Online regex tester with syntax highlighting for PHP/PCRE, Python, Golang, JavaScript. Contextual help, regex quiz, cheat sheet, and community patterns.
Python regex extract
Extract part of a regex match, Use ( ) in regexp and group(1) in python to retrieve the captured string ( re.search will return None if it doesn't find the result, so don't use Regular Expressions in Python Regular expression (RegEx) is an extremely powerful tool for processing and extracting character patterns from text. Regular Expressions are fast and helps you to
Python RegEx: re.match(), re.search(), re.findall() with , What is Regular Expression in Python? A Regular Expression (RE) in a programming language is a special text string used for describing a search pattern. It is extremely useful for extracting information from text such as code, files, log, spreadsheets or even documents. Use in regexp and group(1) in python to retrieve the captured string (re.search will return None if it doesn't find the result, so don't use group() directly): title_search = re.search('<title>(.*)</title>', html, re.IGNORECASE) if title_search: title = title_search.group(1)
Extracting Words from a string in Python using the “re” module, Regular expression (RegEx) is an extremely powerful tool for processing and extracting character patterns from text. Regular Expressions are How to extract data from a string with Python Regular Expressions? Python Server Side Programming Programming The following code extracts data like first_id, second_id, category from given strings
Python regex cheat sheet
Python Regular Expression Cheatsheet, Python Regex Cheatsheet. Regular Expression Basics . Any character except newline. a, The character a. ab, The string ab. a|b, a or b. a*, 0 or more a's. Python Python Regex Cheatsheet. Regular Expression Basics. Any character except newline Regular Expression Flags; i: Ignore case: m ^ and $ match start and end of
Python Regex Cheat Sheet: Regular Expressions in Python, Keep this regex cheat sheet for Python nearby anytime you need to use regular expressions for your data science work, as a quick, handy Python Regex Cheat Sheet. Regex or Regular Expressions are an important part of Python Programming or any other Programming Language. It is used for searching and even replacing the specified text pattern. In the regular expression, a set of characters together form the search pattern. It is also known as reg-ex pattern.
Python regular expression cheatsheet and , Python regular expression cheatsheet and examples. 2020-07-03. pyregex example. Above visualization is a screenshot created using debuggex for the pattern Python RegEx Cheatsheet with Examples Quantifiers match m to n occurrences, but as few as possible (eg., py{1,3}?) {m,n} match m to infinite occurrences (eg., py{3,}) {m,} match from 0 to n occurrences (eg., py{,3}) {,n} match from m to n occurrences (eg., py{1,3}) {m,n} match exactly m occurrences (eg., py{3}) {m} match 0 or 1 occurrences (eg., py?)?
Python string contains regex
Python RegEx, RegEx can be used to check if a string contains the specified search pattern. RegEx Module. Python has a built-in package called re , which can be used to work 1. Here's a function that does what you want: import re def is_match (regex, text): pattern = re.compile (regex, text) return pattern.search (text) is not None. The regular expression search method returns an object on success and None if the pattern is not found in the string.
python's re: return True if string contains regex pattern, import re word = 'fubar' regexp = re.compile(r'ba[rzd]') if regexp.search(word): print 'matched'. RegEx in Python. When you have imported the re module, you can start using regular expressions: Example. Search the string to see if it starts with 'The' and ends with 'Spain': import re. txt = 'The rain in Spain'. x = re.search ('^The.*Spain$', txt) Try it Yourself ».
Python: Check if String Contains Substring, 4) Regular Expressions (REGEX). Regular expressions provide a more flexible (albeit more complex) way to check strings for pattern matching. Python is shipped The solution is to use Python’s raw string notation for regular expressions; backslashes are not handled in any special way in a string literal prefixed with 'r', so r' ' is a two-character string containing ' and 'n', while ' ' is a one-character string containing a newline. Regular expressions will often be written in Python code using this raw string notation.
Which of these options correspond to match the sequence xyz?
Module 1 Quiz Flashcards, [^xyz]. Which of these options correspond to match one of the characters x,y,z? [xyz]. Which of these options correspond to match the sequence xyz? xyz [xyz] [x|y|z] If any? Both [] and | specify alternatives. Following code prints the exact same results: String string = 'the x and the y and the z and the nothing'; evaluatePattern(Pattern.compile('w*[xyz]w*'), string); evaluatePattern(Pattern.compile('w*[x|y|z]w*'), string);
Ultimate Regex Cheat Sheet, These expressions can be used for matching a string of text, find and Used to match 0 or more of the previous (e.g. xy*z could correspond to An ARE can begin with embedded options: a sequence (?xyz) (where xyz is one or more alphabetic characters) specifies options affecting the rest of the RE. These options override any previously determined options — in particular, they can override the case-sensitivity behavior implied by a regex operator, or the flags parameter to a regex
Python Tutorial: Regular Expression, If we match 'cats' in a string that might be still okay, but what about all those for the character class 'any lower case or uppercase letter' [A-Za-z] digits and the underscore, corresponding to the following regular expression: r'[a-zA-Z0-9_]' While the other sequences match characters, - e.g. w matches characters like Match anywhere: By default, a regular expression matches a substring anywhere inside the string to be searched. For example, the regular expression abc matches abc 123, 123 abc, and 123 abc xyz. To require the match to occur only at the beginning or end, use an anchor.
Groupdict regex python
re.MatchObject.groupdict () function in Python – Regex Last Updated : 29 Aug, 2020 This method returns a dictionary with the groupname as keys and the matched string as the value for that key.
groupdict in regex. Browse other questions tagged python python-3.x regex regex-group python-regex or ask your own Converting user input string to regular
A groupdict () expression returns a dictionary containing all the named subgroups of the match, keyed by the subgroup name.
Python regex tester
Pythex: a Python regular expression editor, Pythex is a real-time regular expression editor for Python, a quick way to test your regular expressions. Today is 2021-02-06. pythex is a quick way to test your Python regular expressions. Try writing one or test the example. Match result: Match captures: Regular expression cheatsheet.
regex101: build, debug and share regex, Online regex tester with syntax highlighting for PHP/PCRE, Python, Golang, JavaScript. Contextual help, quiz, cheat sheet, and searchable community patterns. PyRegex is a online regular expression tester to check validity of regular expressions in the Python language regex subset.
Debuggex: Online visual regex tester. JavaScript, Python, and PCRE., Test your regex by visualizing it with a live editor. JavaScript, Python, and PCRE. Online regex tester with syntax highlighting for PHP/PCRE, Python, Golang, JavaScript. Contextual help, regex quiz, cheat sheet, and community patterns.
Error processing SSI filePython starts with regex
Python RegEx, Print the position (start- and end-position) of the first match occurrence. The regular expression looks for any words that starts with an upper case 'S': import re Summary: Can You Use a Regular Expression with the Python startswith Method? No, you cannot use a regular expression with the Python startswith function. But you can use the Python regular expression module re instead. It’s as simple as calling the function match(s1, s2). This finds the regular expression s1 in the string s2. Python Startswith() List
How can I match the start and end in Python's regex?, re.match will match the string at the beginning, in contrast to re.search : re.match(r'(ftp|http)://.*.(jpg|png)$', s). Two things to note here: r' is used Python has a module named re to work with RegEx. Here's an example: import re pattern = '^as$' test_string = 'abyss' result = re.match (pattern, test_string) if result: print('Search successful.') else: print('Search unsuccessful.') Here, we used re.match () function to search pattern within the test_string.
re — Regular expression operations, Patterns which start with negative lookbehind assertions may match at the beginning of the string being searched. (?(id/name)yes-pattern|no-pattern). Will try to RegEx in Python. When you have imported the re module, you can start using regular expressions: Example. Search the string to see if it starts with 'The' and ends with 'Spain': import re. txt = 'The rain in Spain'. x = re.search ('^The.*Spain$', txt) Try it Yourself ».
Error processing SSI filePython split regex
re — Regular expression operations, If maxsplit is nonzero, at most maxsplit splits occur, and the remainder of the string is returned as the final element of the list. >>> >>> re.split(r Regular expression 'd+' would match one or more decimal digits. In this example, we will use this regular expression to split a string into chunks which are separated by one or more decimal digits. Python Program. import re #a string str = 'foo635bar4125mango2apple21orange' #split with regular expression chunks = re.split('d+',str) print(chunks) Run
Split strings in Python (delimiter, line break, regex, etc.), If you want to split a string that matches a regular expression instead of perfect match, use the split() of the re module. In re. split() , specify the regular expression pattern in the first parameter and the target character string in the second parameter. You could use a lookahead: re.split (r' [ ] (?= [A-Z]+b)', input) This will split at every space that is followed by a string of upper-case letters which end in a word-boundary. Note that the square brackets are only for readability and could as well be omitted.
Split string based on regex, What is the best way to split a string like 'HELLO there HOW are YOU' by upper case words (in Python)?. So I'd end up with an array like such: Let us see how to split a string using regex in python. We can use re.split () for the same. re is the module and split () is the inbuilt method in that module. For example. import re myvar = 'sky1cloud3blue333red' print (re.split ('d+', myvar)) Note: Make sure to import the re module or else it will not work.
Error processing SSI fileRe sub Python 3
re — Regular expression operations, For example, a{3,5} will match from 3 to 5 'a' characters. Omitting m specifies a lower in a string passed to the repl argument of re.sub(). g<quote>. g<1>. 1 re.sub (pattern, repl, string, count=0, flags=0) ¶ Return the string obtained by replacing the leftmost non-overlapping occurrences of pattern in string by the replacement repl. If the pattern isn’t found, string is returned unchanged. repl can be a string or a function; if it is a string, any backslash escapes in it are processed.
Python re.sub Examples, If you want to replace a string that matches a regular expression instead of perfect match, use the sub() of the re module. In re. sub() , specify a regular expression pattern in the first argument, a new string in the second argument, and a string to be processed in the third argument. re functions in python; re.sub python 3; python b regex; re module grants all regular expression functions to Python; re.sub return type; python regex for; python libraty re; re.verbose; re.sub() python 3; python regex fetch eager; python 're.search' reg : str in python; string pattern match python; re.compile(regex) relative match python; python 're.sub' re . split
Python Cheat Sheet Pdf
Replace strings in Python (replace, translate, re.sub, re.subn), Syntax. re.sub(pattern, repl, string, max=0). This method replaces all occurrences of the RE pattern in string with text = 'file1 file2 file3' findallfile = re.findall(r'filedb', text) for instance in findallfile: textwithzeros = re.sub('file', 'file0', text) 'textwithzeros' should now be a new version of the 'text' string with '0' before each number. Try it out!
Error processing SSI filePython 3 Regex Cheat Sheet Download
More Articles
